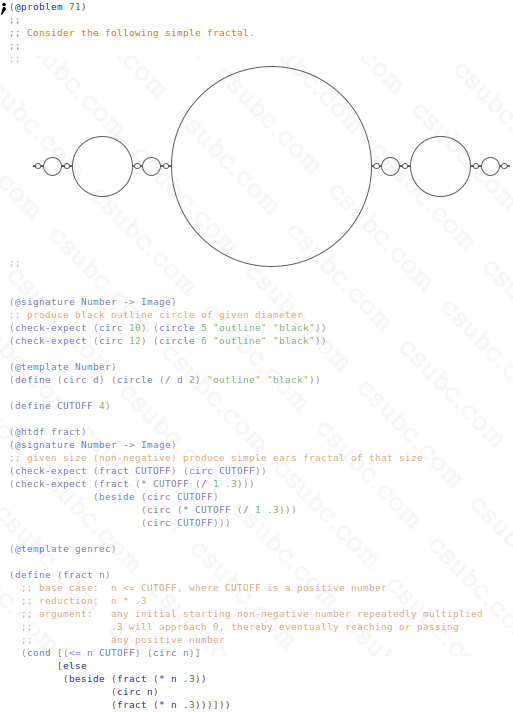
(require spd/tags)
(require 2htdp/image)
(@signature Number -> Image)
;; produce black outline circle of given diameter
(check-expect (circ 10) (circle 5 "outline" "black"))
(check-expect (circ 12) (circle 6 "outline" "black"))
(define (circ d)
(circle (/ d 2) "outline" "black"))
(define CUTOFF 4)
(@htdf fract)
(@signature Number -> Image)
;given size (non-negative) produce simple ears fractal of that size
(check-expect (fract CUTOFF) (circ CUTOFF))
(check-expect (fract (* CUTOFF (/ 1 .3)))
(beside (circ CUTOFF)
(circ (* CUTOFF (/ 1 .3)))
(circ CUTOFF)))
(@template-origin genrec)
(define (fract n) empty-image) ;stub
-
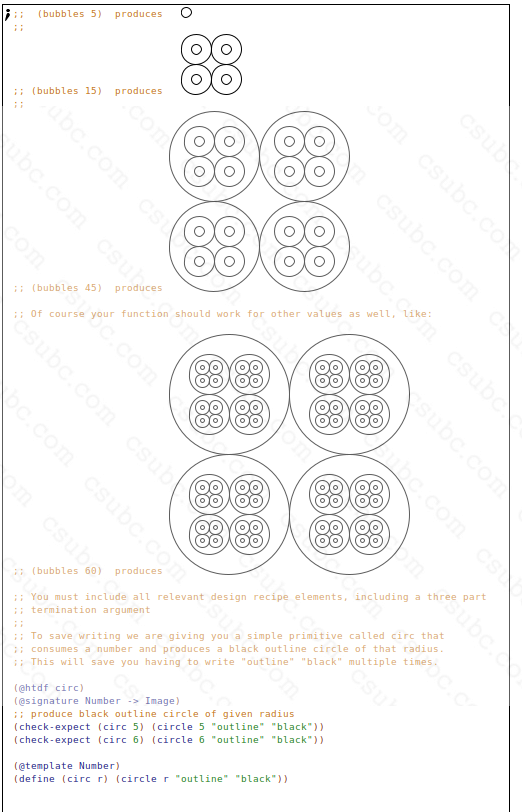
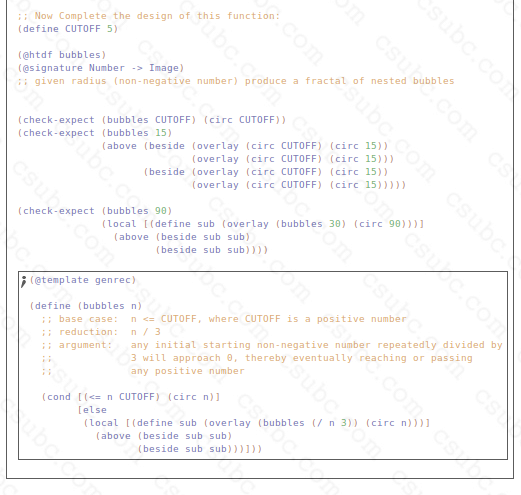
(require spd/tags)
(require 2htdp/image)
;; You must include all relevant design recipe elements, including a three part
;; termination argument
;; To save writing we are giving you a simple primitive called circ that
;; consumes a number and produces a black outline circle of that radius.
;; This will save you having to write "outline" "black" multiple times.
(@htdf circ)
(@signature Number -> Image)
;; produce black outline circle of given radius
(check-expect (circ 5)(circle 5 "outline" "black"))
(check-expect (circ 6)(circle 6 "outline" "black"))
(@template-origin Number)
(define (circ r)
(circle r "outline" "black"))
;; Now Complete the design of this function:
(define CUTOFF 5)
(@htdf bubbles)
(@signature Number -> Image)
;; given radius (non-negative number) produce a fractal of nested bubbles
(check-expect (bubbles CUTOFF) (circ CUTOFF))
(check-expect (bubbles 15)
(above (beside (overlay (circ CUTOFF) (circ 15))
(overlay (circ CUTOFF) (circ 15)))
(beside (overlay (circ CUTOFF) (circ 15))
(overlay (circ CUTOFF) (circ 15)))))
(check-expect (bubbles 90)
(local [(define sub (overlay (bubbles 30) (circ 90)))]
(above (beside sub sub)
(beside sub sub))))
(define (bubbles n) empty-image) ;stub
;; Comment out stub and finish bubbles below