abstraction就是把本来很容易理解的东西变成很抽象的意思

abstraction分三部分
1. abstract 两个相似的function
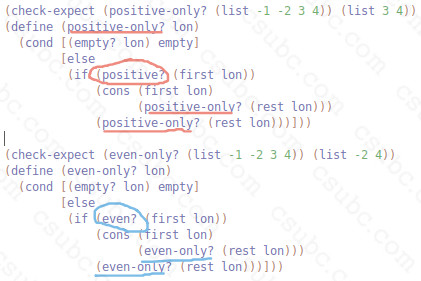
两个function相同位置但不同的内容,我们可以统一替换并abstract出一个function
even? 和 positive? 可以加多一个参数fn表示,self reference的部分叫 only?
再用这个only? 反写positive-only? 和 even-only?
(define (only? fn lon)
(cond [(empty? lon) empty]
[else
(if (fn (first lon))
(cons (first lon)
(only? (rest lon)))
(only? (rest lon)))]))
(define (positive-only? lon)
(only? positive? lon))
(define (even-only? lon)
(only? even? lon))
aaaaaa
2. build-in abstract function for list operation
;; Natural (Natural -> X) -> (listof X)
;; produces (list (f 0) ... (f (- n 1)))
;; (define (build-list n f) ...)
(build-list 5 identity) -> (list 0 1 2 3 4)
(build-list 5 add1) -> (list 1 2 3 4 5)
;; (X -> boolean) (listof X) -> (listof X)
;; produce a list from all those items on lox for which p holds
;; (define (filter p lox) ...)
(filter positive? (list 1 -2 3 4 -5)) -> (list 1 3 4)
(filter odd? (list 1 -2 3 4 -5)) -> (list 1 3 -5)
;; (X -> Y) (listof X) -> (listof Y)
;; produce a list by applying f to each item on lox
;; that is, (map f (list x-1 ... x-n)) = (list (f x-1) ... (f x-n))
;; (define (map f lox) ...)
(map add1 (list 1 2 3 4 5)) -> (list 2 3 4 5 6)
(map string-length (list "a" "aa" "aaa")) -> (list 1 2 3)
;; (X -> boolean) (listof X) -> boolean
;; produce true if p produces true for every element of lox
;; (define (andmap p lox) ...)
(andmap positive? (list 1 2 3)) -> true
(andmap positive? (list 1 -2 3)) -> false
;; (X -> boolean) (listof X) -> boolean
;; produce true if p produces true for some element of lox
;; (define (ormap p lox) ...)
(ormap positive? (list 1 -2 3)) -> true
(ormap positive? (list -1 -2 -3)) -> false
;; (X Y -> Y) Y (listof X) -> Y
;; (foldr f base (list x-1 ... x-n)) = (f x-1 ... (f x-n base))
;; (define (foldr f base lox) ...)
(foldr + 0 (list 1 2 3)) -> 6
(foldr string-append "" (list "a" "b" "c")) -> "abc"
;; (X Y -> Y) Y (listof X) -> Y
;; (foldl f base (list x-1 ... x-n)) = (f x-n ... (f x-1 base))
;; (define (foldl f base lox) ...)
(foldl + 0 (list 1 2 3)) -> 6
(foldl string-append "" (list "a" "b" "c")) -> "cba"
3. closure
(filter positive? (list 1 -2 3 4 -5)) -> (list 1 3 4)
这里我们想保留list里面的正数,positive? 是racket自带的,可以直接用。
假如我们想保留list里面> 5的数, 而racket并没有判断是否>5的自带function,
这时我们需要用local提供一个helper
(local [(define (fn n) (> n 5))]
(filter fn (list 1 -2 3 4 -5)))
这个形式就叫closure
4.fold-function
注意这个fold-function跟foldl和foldr没一点关系
如果上面filter, andmap, ormap, foldl,foldr什么的是racket自带的built-in abstracet function
fold-function的意思要尼自己写一个abstracet function,来让别人用。
一般是跟mutual reference结合
具体看edx视频
内容太长,估计没个800来字,写不完。。。
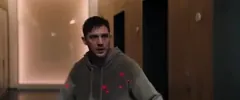
但是有关于fold-function问题的话,可以在下面post。