Admin
===============================Q1===============================
For each of the five following subproblems, determine whether the code has a memory leak,
dangling pointer, or is poorly structured and then indicate what change(s) are required to fix
this problem (if there is one).
a) [2]
char* makeRandomKey(int bytes) {
char *c = malloc(bytes);
for (int i=0; i<bytes; i++)
c[i] = rand();
return c;
}
long getKey() {
char *key = makeRandomKey(8);
long l = *((long *) key);
free(key);
return l;
}
Problem:
(1)Dangling Pointer (2)Memory Leak (3)Both (4)No bug; but poor structure (5)No problem
Change:
(1)Add malloc (2)Move malloc (3)Add free (4)Move free (5)No changes (6)Other changes
(7)Add reference counting
b) [2]
char* makeRandomKey(char *c, int bytes) {
for (int i=0; i<bytes; i++)
c[i] = rand();
return c;
}
long getKey() {
char *key = malloc(8);
makeRandomKey(key, 8);
long l = *((long *) key);
free(key);
return l;
}
Problem:
(1)Dangling Pointer (2)Memory Leak (3)Both (4)No bug; but poor structure (5)No problem
Change:
(1)Add malloc (2)Move malloc (3)Add free (4)Move free (5)No changes (6)Other changes
(7)Add reference counting
c) [2]
char* makeRandomKey(char *c, int bytes) {
for (int i=0; i<bytes; i++)
c[i] = rand();
return c;
}
int unlock(char* key) {
// we do not know what this does ...
}
int getKeyAndTryUnlock() {
char *key = malloc(8);
makeRandomKey(key, 8);
int unlocked = unlock(key);
free(key);
return unlocked;
}
Problem:
(1)Dangling Pointer (2)Memory Leak (3)Both (4)No bug; but poor structure (5)No problem
Change:
(1)Add malloc (2)Move malloc (3)Add free (4)Move free (5)No changes (6)Other changes
(7)Add reference counting
d) [2]
char* makeRandomKey(int bytes) {
char c[bytes];
for (int i=0; i<bytes; i++)
c[i] = rand();
return c;
}
long getKey() {
char* key = makeRandomKey(8);
return *((long *) key);
}
Problem:
(1)Dangling Pointer (2)Memory Leak (3)Both (4)No bug; but poor structure (5)No problem
Change:
(1)Add malloc (2)Move malloc (3)Add free (4)Move free (5)No changes (6)Other changes
(7)Add reference counting
e) [2]
char* makeRandomKey(int bytes) {
char *c = malloc(bytes);
for (int i=0; i<bytes; i++)
c[i] = rand();
return c;
}
long getKey() {
char* key = makeRandomKey(8);
return *((long *) key);
}
Problem:
(1)Dangling Pointer (2)Memory Leak (3)Both (4)No bug; but poor structure (5)No problem
Change:
(1)Add malloc (2)Move malloc (3)Add free (4)Move free (5)No changes (6)Other changes
(7)Add reference counting
===============================Q2===============================
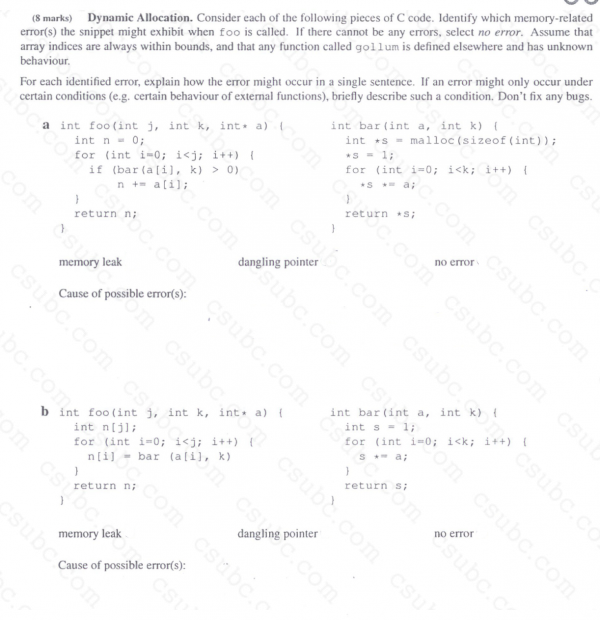
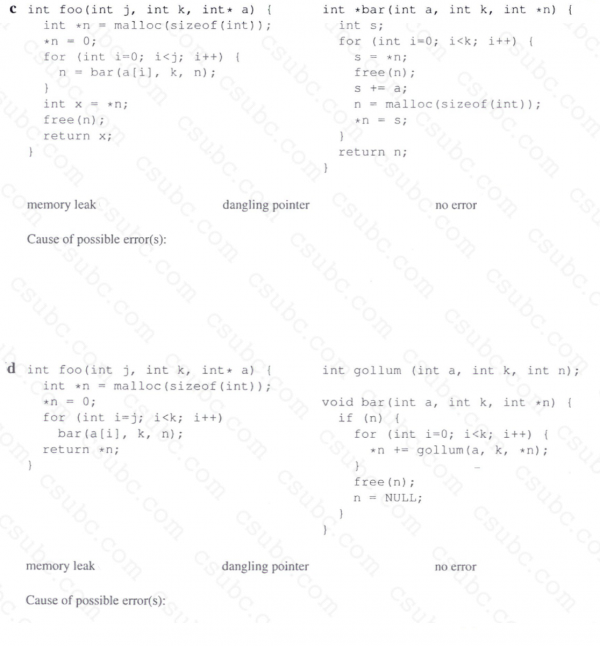
===============================Q3===============================
===============================Q4===============================
===============================Q5===============================
===============================Q6===============================
===============================Q7===============================
===============================Q8===============================
===============================Q9===============================
===============================Q10===============================
===============================Q11===============================
===============================Q12===============================