Admin Access
===========Q1(Winter 2019 Term 1)===========
Numbers and Memory [8 marks]
a) Consider the following code running on a little-endian machine where
the address of i is 0x1000.
int i = 0x1234;
What is the hex value of the byte at address 0x1000?
b) Consider the following code
int i = 0x56789a;
char c = (char) i;
int j = c;
What is the hex value of c ?
What is the hex value of j ?
c)
Following the execution of these 3 lines of code on a Little-Endian machine,
what is the value of the c?
int i = 0x12345678;
char *cp = (char*) &i;
char c = cp[0];
d)
Following the execution of these two lines of code, what is the value of i?
char c = 0xff;
int i = c;
e)
Following the execution of these two lines of code, what is the value of d?
char c = 0x84;
char d = c >> 1;
f)
Following the execution of these two lines of code, what is the value of d?
char c = 0x73;
char d = c & 0xf;
===========Q2(Winter 2018, Term 2)===========
Memory and Numbers. Consider the execution of the following code on a little-endian processor.
Assume that the address of i is 0x1000 and that the compiler allocates the three global
variables contiguously,in the order they appear, wasting no memory between them other
than what is required to ensure that they are properly aligned (and assuming that char’s
are signed, and int’s and pointers are 4-bytes long).
int i;
char c;
int* j
void foo() {
i = 0x22446688;
j = &i;
c = (char) i;
*j = i | c;
}
For every byte of memory whose value you cand etermine from the information given above,
give its value in hex on the line labeled with its address.
For addresses whose value you can not determine, check the Unknown box.
0x1000: Unknown_ or Value ____________
0x1001: Unknown_ or Value ____________
0x1002: Unknown_ or Value ____________
0x1003: Unknown_ or Value ____________
0x1004: Unknown_ or Value ____________
0x1005: Unknown_ or Value ____________
0x1006: Unknown_ or Value ____________
0x1007: Unknown_ or Value ____________
0x1008: Unknown_ or Value ____________
0x1009: Unknown_ or Value ____________
0x100a: Unknown_ or Value ____________
0x100b: Unknown_ or Value ____________
===========Q3(Winter 2019 Term 2)===========
Memory and Numbers.
Consider the execution of the following code. Assume that the compiler
has statically allocated p to start at address 0x2020, and consecutively
allocates the subsequent variables, inserting padding (wasting memory)
only where needed to ensure that each variable is aligned.
Assume too that char’s are signed, and int’s and pointers are 4 bytes long.
Prior to the execution of foo(), assume that the memory contents are
indeterminate (i.e. anything could hold any value).
int *p; void foo() {
int i; p=&i;
char c; i = 0x0badcafe;
short s; c = (char)p;
s = (short)i;
*p = *p | s;
}
Assuming that the code above executes on a little-endian machine,
give the value in hex stored at each memory address below,
or write UNKNOWN if the value cannot be determined completely.
0x2020: ____________
0x2021: ____________
0x2022: ____________
0x2023: ____________
0x2024: ____________
0x2025: ____________
0x2026: ____________
0x2027: ____________
0x2028: ____________
0x2029: ____________
0x202a: ____________
0x202b: ____________
===========Q4(Winter 2021)===========
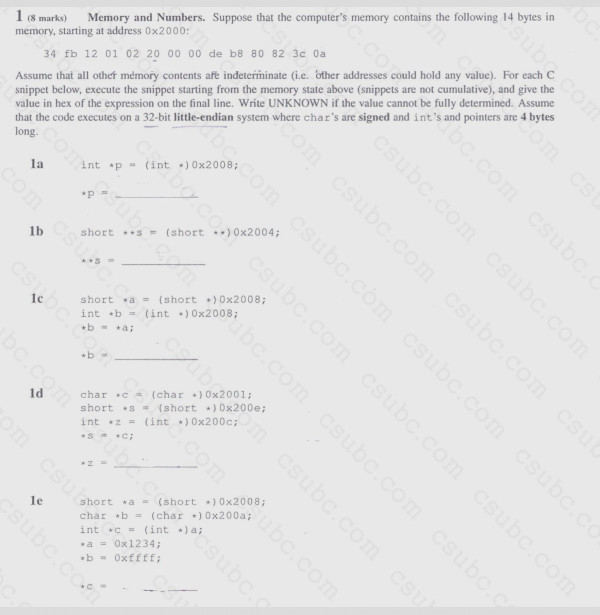